First we will start with cleaning up our application which we created at first post. We will not use about, contact and tabs pages so let's delete about, contact and tabs folders under pages folder, leaving only home page folder:

Delete AboutPage, ContactPage and TabsPage references from app.module.ts. Final version should look like this:
import { NgModule } from '@angular/core'; import { IonicApp, IonicModule } from 'ionic-angular'; import { MyApp } from './app.component'; import { HomePage } from '../pages/home/home'; @NgModule({ declarations: [ MyApp, HomePage ], imports: [ IonicModule.forRoot(MyApp) ], bootstrap: [IonicApp], entryComponents: [ MyApp, HomePage ], providers: [] }) export class AppModule { }
Remove TabsPage reference from app.component.cs and import HomePage. Set rootPage to HomePage:
import { Component } from '@angular/core'; import { Platform } from 'ionic-angular'; import { StatusBar } from 'ionic-native'; import { HomePage } from '../pages/home/home'; @Component({ template: `<ion-nav [root]="rootPage"></ion-nav>` }) export class MyApp { rootPage = HomePage; constructor(platform: Platform) { platform.ready().then(() => { // Okay, so the platform is ready and our plugins are available. // Here you can do any higher level native things you might need. StatusBar.styleDefault(); }); } }
We finally removed unnecessary pages in our application. Now we will add some images to our application. In an Ionic application images and other sources are put under src/assets folder. Let's create an img folder under src/assets folder and put some images inside it:
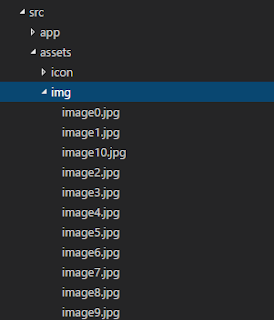
Create an image.ts file under app folder and define and Image class inside it:
export class Image { id: number; path: string; }
Create an images.ts file under app folder and define an Image array which contains our image path and id values:
import { Image } from "./image"; export const IMAGES: Image[] = [ { id: 0, path: "assets/img/image0.jpg"}, { id: 1, path: "assets/img/image1.jpg"}, { id: 2, path: "assets/img/image2.jpg"}, { id: 3, path: "assets/img/image3.jpg"}, { id: 4, path: "assets/img/image4.jpg"}, { id: 5, path: "assets/img/image5.jpg"}, { id: 6, path: "assets/img/image6.jpg"}, { id: 7, path: "assets/img/image7.jpg"}, { id: 8, path: "assets/img/image8.jpg"}, { id: 9, path: "assets/img/image9.jpg"}, { id: 10, path: "assets/img/image10.jpg"} ];
Create an image.service.ts file under app folder and implement ImageService to return our images:
import { Injectable } from '@angular/core'; import { Image } from './Image'; import { IMAGES } from './Images'; @Injectable() export class ImageService { getImages(): Promise<Image[]> { return Promise.resolve(IMAGES); } }
Put an ion-grid in home.html and bind each of our images to grid cells using Angular 2's *ngFor directive. We will show three images at each row so we will use ion-row's wrap attribute and width-33 as relative width. You can change width to show different numbers of images, for example width-50 to show two images in each row or width-25 for four images etc:
<ion-content> <ion-grid> <ion-row wrap> <ion-col width-33 *ngFor="let image of images"> <img [src]="image.path"> </ion-col> </ion-row> </ion-grid> </ion-content>
Import ImageService in home.ts to use it. Specify it as a provider for HomePage component and get images by calling ImageService and set return value to component's images property:
import { Component, ViewChild, OnInit } from '@angular/core'; import { NavController } from 'ionic-angular'; import { SlidePage } from '../slide/slide'; import {Image} from '../../image'; import { ImageService } from '../../image.service'; @Component({ templateUrl: 'build/pages/home/home.html', providers: [ImageService] }) export class HomePage implements OnInit { images: Image[]; constructor(public navCtrl: NavController, private imageService: ImageService) { } ngOnInit(): void { this.imageService.getImages().then(images => this.images = images); } }
Last of all, change grid background color in home.scss and then our image gallery is ready:
ion-grid { background-color: #0e1717; }
Thank you Hakan Macat for your post. It works perfectly!
ReplyDeleteThis comment has been removed by a blog administrator.
ReplyDelete